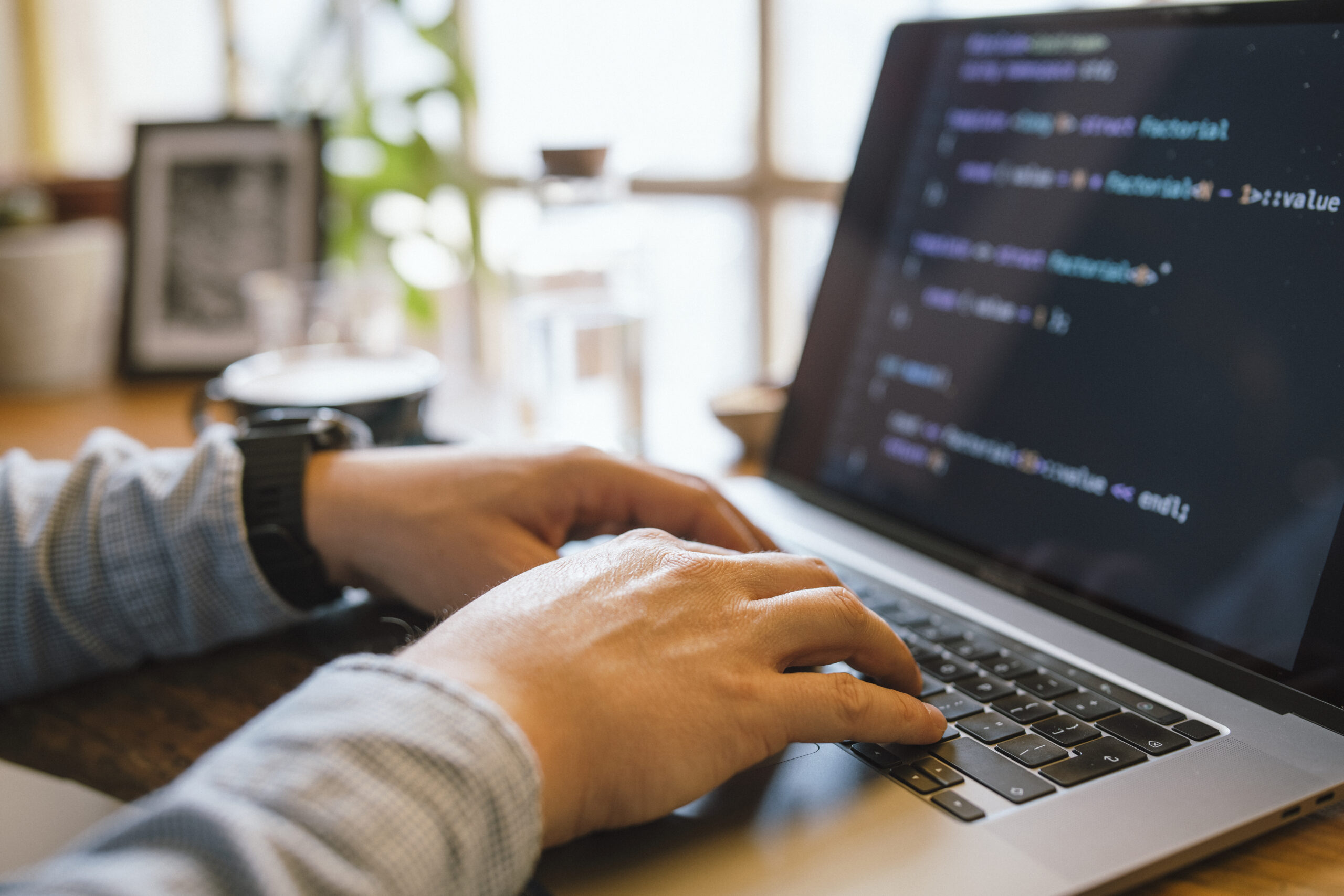
Debugging is The most essential — nevertheless typically forgotten — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly boost your productivity. Listed here are many approaches to help you developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
Among the list of quickest techniques developers can elevate their debugging competencies is by mastering the instruments they use every single day. Although writing code is a person Component of growth, realizing how you can connect with it properly in the course of execution is equally significant. Present day improvement environments come Geared up with effective debugging capabilities — but quite a few developers only scratch the area of what these equipment can do.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources allow you to established breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code within the fly. When used effectively, they let you notice precisely how your code behaves through execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, look at serious-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable jobs.
For backend or system-degree builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Command more than managing processes and memory administration. Learning these instruments may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Handle programs like Git to be familiar with code history, locate the exact minute bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools implies heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your advancement natural environment to make sure that when challenges crop up, you’re not lost at midnight. The better you realize your resources, the more time you can spend solving the particular problem rather then fumbling as a result of the procedure.
Reproduce the situation
One of the more important — and sometimes neglected — methods in successful debugging is reproducing the situation. In advance of leaping in to the code or creating guesses, developers want to create a dependable natural environment or circumstance in which the bug reliably appears. Without the need of reproducibility, correcting a bug gets a sport of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is gathering just as much context as you can. Inquire questions like: What steps led to The difficulty? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater element you've got, the easier it will become to isolate the exact disorders beneath which the bug takes place.
As soon as you’ve gathered ample info, try to recreate the challenge in your local natural environment. This could necessarily mean inputting the identical details, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the sting instances or condition transitions associated. These assessments not only support expose the condition but additionally protect against regressions in the future.
Often, The difficulty might be setting-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t just a stage — it’s a attitude. It calls for tolerance, observation, in addition to a methodical strategy. But as soon as you can continuously recreate the bug, you might be presently halfway to repairing it. By using a reproducible circumstance, You may use your debugging applications more effectively, test possible fixes safely, and communicate much more clearly together with your group or customers. It turns an abstract complaint right into a concrete obstacle — Which’s the place developers thrive.
Study and Comprehend the Error Messages
Mistake messages in many cases are the most valuable clues a developer has when something goes wrong. Rather then looking at them as discouraging interruptions, developers ought to discover to take care of mistake messages as direct communications in the system. They normally inform you what exactly occurred, where it transpired, and often even why it occurred — if you know the way to interpret them.
Start out by looking through the message diligently As well as in complete. Many builders, especially when less than time strain, glance at the main line and immediately get started generating assumptions. But deeper from the error stack or logs may perhaps lie the real root cause. Don’t just duplicate and paste error messages into search engines like google — browse and realize them initial.
Crack the error down into sections. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Will it level to a selected file and line variety? What module or function brought on it? These concerns can guideline your investigation and level you towards the accountable code.
It’s also practical to comprehend the terminology from the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Finding out to recognize these can substantially increase your debugging method.
Some glitches are vague or generic, and in People cases, it’s critical to look at the context by which the error transpired. Look at associated log entries, input values, and up to date variations within the codebase.
Don’t forget compiler or linter warnings possibly. These frequently precede greater difficulties and supply hints about potential bugs.
Eventually, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them properly turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and turn into a additional economical and confident developer.
Use Logging Wisely
Logging is Probably the most effective instruments in a very developer’s debugging toolkit. When made use of effectively, it offers real-time insights into how an application behaves, helping you understand what’s happening under the hood without needing to pause execution or step through the code line by line.
A great logging technique starts with understanding what to log and at what level. Typical logging amounts contain DEBUG, Information, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic info throughout improvement, INFO for general situations (like effective start-ups), Alert for probable troubles that don’t break the application, Mistake for true difficulties, and FATAL when the procedure can’t keep on.
Keep away from flooding your logs with extreme or irrelevant information. Too much logging can obscure vital messages and decelerate your method. Focus on critical functions, state variations, input/output values, and critical conclusion factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in production environments wherever stepping via code isn’t doable.
Additionally, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, sensible logging is about harmony and clarity. Which has a effectively-considered-out logging method, it is possible to lessen the time it will take to identify challenges, acquire further visibility into your purposes, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently discover and take care of bugs, builders should strategy the method similar to a detective resolving a secret. This mindset aids break check here down intricate challenges into workable parts and comply with clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality difficulties. Identical to a detective surveys against the law scene, obtain just as much applicable data as you may without the need of leaping to conclusions. Use logs, exam conditions, and person experiences to piece alongside one another a transparent photo of what’s occurring.
Following, kind hypotheses. Question by yourself: What could possibly be triggering this conduct? Have any adjustments not too long ago been created towards the codebase? Has this problem occurred before less than similar instances? The target is usually to slim down choices and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble inside a managed natural environment. In case you suspect a selected operate or component, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code inquiries and Allow the results direct you closer to the reality.
Spend shut consideration to little aspects. Bugs typically hide from the least envisioned areas—similar to a missing semicolon, an off-by-one mistake, or perhaps a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without entirely knowing it. Non permanent fixes might disguise the real challenge, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run issues and support Many others realize your reasoning.
By imagining just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and become more effective at uncovering hidden difficulties in elaborate methods.
Publish Assessments
Crafting tests is one of the best solutions to improve your debugging abilities and All round progress performance. Checks not only assist catch bugs early but in addition serve as a security Internet that provides you self esteem when earning changes for your codebase. A effectively-examined application is simpler to debug since it means that you can pinpoint accurately where and when a problem occurs.
Get started with device checks, which deal with unique capabilities or modules. These compact, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as predicted. Each time a examination fails, you right away know exactly where to appear, considerably reducing some time expended debugging. Device exams are Particularly helpful for catching regression bugs—problems that reappear after Beforehand staying mounted.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These assistance be sure that a variety of areas of your application do the job jointly easily. They’re particularly helpful for catching bugs that manifest in intricate methods with multiple parts or providers interacting. If something breaks, your assessments can tell you which Element of the pipeline failed and less than what problems.
Creating checks also forces you to Assume critically about your code. To check a function adequately, you may need to understand its inputs, predicted outputs, and edge instances. This standard of comprehending Obviously prospects to higher code structure and less bugs.
When debugging a difficulty, writing a failing take a look at that reproduces the bug can be a strong starting point. After the exam fails constantly, you may concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy makes sure that the same bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you catch a lot more bugs, more rapidly plus more reliably.
Take Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the condition—staring at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking evident problems or misreading code that you just wrote just hrs previously. Within this state, your Mind results in being fewer economical at challenge-fixing. A short walk, a espresso crack, or maybe switching to another undertaking for 10–15 minutes can refresh your focus. Many builders report obtaining the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, Particularly all through extended debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Vitality and a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do something unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, but it essentially leads to more rapidly and more effective debugging Over time.
Briefly, taking breaks will not be a sign of weakness—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and assists you stay away from the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Each individual Bug
Each bug you face is a lot more than simply a temporary setback—It really is a possibility to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing valuable in the event you make time to mirror and assess what went Completely wrong.
Start by asking your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better methods like unit testing, code reviews, or logging? The answers often reveal blind places in the workflow or understanding and help you build stronger coding habits moving forward.
Documenting bugs can be a superb behavior. Preserve a developer journal or preserve a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring issues or popular faults—which you could proactively keep away from.
In crew environments, sharing Everything you've discovered from the bug with the peers may be especially highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, aiding others steer clear of the identical issue boosts staff effectiveness and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll get started appreciating them as vital parts of your progress journey. In any case, a lot of the ideal builders usually are not those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills usually takes time, apply, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you happen to be knee-deep in a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.